Assertions are very important part of test automation. It helps to validate certain conditions to be verified. The term “assert” in programming and software testing refers to a statement or function used to validate an assumption or condition within a program. In Selenium Webdriver Assertions are way to confirm that certain conditions are true during the execution of the code.
When an assertion is made, it checks whether a given condition holds true. If the condition is true, the program continues execution without any interruption. However, if the condition evaluates to false, the assertion fails. This failure typically results in an error or an exception, halting the program’s execution or indicating a problem in the code that needs attention.
Assertions are checkpoints within your test scripts that verify whether an expected result matches the actual result. They serve as guardians, validating that the application behaves as intended.
Types of Selenium Webdriver Assertions – TestNG
There are two types of assertions:
- Hard Assertions
- Soft Assertions Or Verification
Based on the need of test case, as tester can decide whether soft assert needs to be added or hard assert is required. Let’s understand what are these asserts and when to use it:
Hard Assert
Hard assertions stop the test execution immediately upon encountering a failure. They’re useful when you want to halt further execution upon the first failure, focusing on fixing the primary issue. Below are different types of hard asserts:
- Boolean Assertions: These verify whether a condition evaluates to true or false. They are the most basic form of assertion, checking for simple conditions like checking if a variable is not null or if a certain value matches an expected outcome.
- assertTrue(calculator.add(2, 3) == 5, "Addition result should be 5");
- Equality Assertions: These compare expected and actual values to check if they are equal. They are often used to verify if a computed or retrieved value matches the anticipated result.
- assertEquals(calculator.divide(10, 2), 5, "Division result should be 5");
- Nullness Assertions: Specifically, these assert whether an object or variable is null or not null. They’re crucial in ensuring that variables have been properly initialized or that functions return valid values.
- assertNotNull(calculator.divide(5, 2), "Division result should not be null");
- Comparison Assertions: These assertions check if a value is greater than, less than, greater than or equal to, or less than or equal to another value. They’re useful for numeric comparisons.
- assertTrue(calculator.divide(10, 2) > 0, "Division result should be greater than 0");
- Collection Assertions: Used when dealing with collections or arrays, these assertions verify the size, presence of elements, or specific elements within collections.
- assertArrayEquals(new int[]{1, 2, 3}, new int[]{1, 2, 3}, "Arrays should be equal");
Soft Assert
Soft Assert: These allow the test to continue after encountering a failure, collecting multiple assertion failures rather than stopping at the first one. It’s beneficial when you want to gather a comprehensive view of all test failures in a single run. After using assertions with Soft assert, assertAll()
is called at the end to report all the failures together after all assertions have been made.
SoftAssert softAssert = new SoftAssert();
// Performing soft assertions
int expectedSum = 5;
int actualSum = 2 + 3;
softAssert.assertEquals(actualSum, expectedSum, "Sum should be 5");
String expectedString = "TestNG";
String actualString = "Selenium";
softAssert.assertEquals(actualString, expectedString, "Strings should match");
boolean condition = false;
softAssert.assertTrue(condition, "Condition should be true");
// Call assertAll to report all failures together
softAssert.assertAll();
Soft Assert Vs Hard Assert
Based on | Hard Asserts | Soft Asserts |
Immediate Termination | The test execution halts immediately, and the control flow stops. | The test execution continues, and all failures are collected without terminating the test. |
Single Failure | Only one failure is reported at a time. The test stops upon encountering the first failed assertion, which might limit insight into other potential issues. | Soft asserts collect all the failures encountered during the test, allowing a comprehensive view of all the issues. |
Implementation | Hard asserts are simpler to implement as they directly use assertion methods like assertEquals , assertTrue , etc. | Soft asserts often require additional handling, such as using a specific library or manually managing the SoftAssert instance to ensure assertAll() is called to report all failures. |
Feedback | Provides less granular feedback as it stops at the first failure, potentially missing other issues that could be revealed by further test runs. | Offers more granular feedback by aggregating all the failures, providing a comprehensive view of multiple issues in a single test run. |
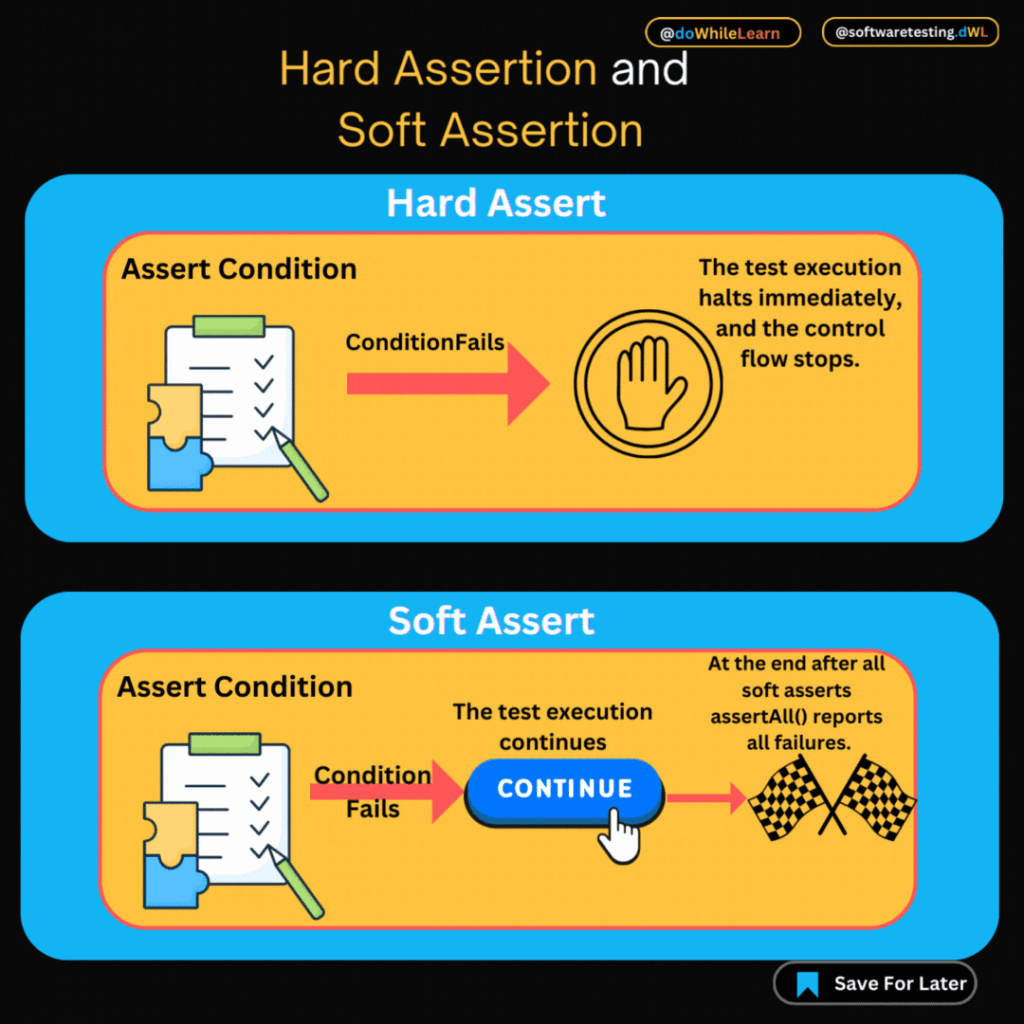
The choice of assertion types depends on the specific scenario and what you’re trying to validate within your code or test cases. Using a combination of these assertion types helps ensure comprehensive validation of the software under test.
Happy learning!